fileExists(filepath); | ||
Parameter | Type | Description |
filepath | string | Path to the file |
Return value | boolean | Returns true if the specified file exists. |
Example
let filestatus = fileExists("c:/example/file.txt");
println(filestatus);
isFileReadable(filepath); | ||
Parameter | Type | Description |
filepath | string | File path |
Return value | boolean | Returns true if the specified file exists and can be read. |
Example
let filestatus = isFileReadable("c:/example/file.txt");
println(filestatus);
isFileWriteable(filepath); | ||
Parameter | Type | Description |
filepath | string | File path |
Return value | boolean | Returns true if the specified file exist and can be written. |
Example
let filestatus = isFileWriteable("c:/example/file.txt");
println(filestatus);
exportArchive(path); | ||||
Parameter | Type | Description | ||
path | string | Path + name of the file | ||
Return value | int | 1 = Export successful -1 = No write permissions to the specified path -2 = Directory could not be created -4 = Export error | ||
Possible errors | The user does not have write permissions to the specified path |
exportNotifications(path); | ||||
Parameter | Type | Description | ||
path | string | Path + name of the file | ||
Possible errors | The user does not have write permissions to the specified path. |
Example 1 Export calculation messages as .xml.
exportNotifications("C:\\temp\\notifications.xml");
isAttrLocked(attrID, compID); | ||
Parameter | Type | Description |
attrID | string | Unique ID of the attribute. |
compID | int | Unique ID of the component in the model. |
Return value | boolean | true - attribute cannot be written. false - attribute can be written. |
In some circumstances, the status of an attribute may be "locked." These attributes cannot be changed via script. For example, this is the case if specification of the attribute value would lead to overdetermination of the model.
Example
If the option for "Specification of addendum modification acc. to DIN 3992" is selected from the distribution of the addendum modification pull-down menu for the cylindrical gear component in the Editor, the attributes for manually specifying the nominal addendum modification coefficient are locked and hidden.
The script checks whether the "addendum modification coefficient" attribute can be written. If so, the new value is set. If not, a message stating "nominal addendum modification coefficient cannot be written, check the distribution of the addendum modification" is shown.
let gearID = 8;
let addendum_modification = 0.3;
if(isAttrLocked("addendum modification coefficient", gearID) == false){
setAttr("addendum modification coefficient", gearID, addendum_modification, EDAT);
}else{
println("Addendum modification factor cannot be set, please check the addendum modification allocation.");
}
delRDAT(); | ||
Parameter | Type | Description |
- | - | - |
For more information see Data integrity (EDAT/RDAT)
Example 1 Delete all RDAT values.
delRDAT();
clearMonitor(); | ||
Parameter | Type | Description |
- | - | - |
Example 1 Clear the monitor
clearMonitor();
stopScript(message); | ||
Parameter | Type | Description |
message | any | Text or data to be output on the Scripting Monitor after termination of the script |
Example 1 Output multiple messages in a loop
1var safety = 1.8;
2if(safety > 2){
3 generateReport("c:\\template.wbrep", "c:\\report.html");
4}else{
5 stopScript("The safety is: "+safety+"\nScript was stopped");
}
| ||
Parameter | Description | |
align | Text direction (left or right justified) | |
width | Field width | |
precision | Number of decimal places to be shown | |
type | Data type of the value to be formatted | |
value | Value to be formatted |
1 | format("%-12s", "Power"); | (-) left justified (12) 12 spaces wide (s) value of type string |
2 | format("%-3s", "P"); | (-) left justified (3) 3 spaces wide (s) value of type string |
3 | format("%10.3f", 148,2456); | () right justified (10) 10 spaces wide (f) value of type floating point number (.3) 3 decimal places |
4 | format("%3s", "kW"); | () right justified (3) 3 spaces wide (s) value of type string |
loadModel(path) | ||
Parameter | Type | Description |
path | string | Path + name of the file |
Possible errors | Model file does not exist or access is denied. |
Note
This function can only be used in batch operation.
Example 1 Load a model from the specified directory.
loadModel("c:\\modell.wbpz");
validateRexsModel(path); | ||||
Parameter | Type | Description | ||
path | string | Path + Name of the REXS file | ||
Return value | object | JSON object with the arrays "errors" and "warnings" | ||
Possible errors | The specified path does not exist. |
Example Validate REXS file and output result on the scripting console
let result = validateRexsModel("c:/example/test.rexs");
println("errors:");
for (let i = 0; i < result.errors.length; i++)
{
println(i + ": "+result.errors[i].error);
}
println(" ");
println("warnings:");
for (let i = 0; i < result.warnings.length; i++){
println(i + ": "+result.warnings[i].warning);
}
startTransactionMode(); |
The following functions are disabled:
3D View updates
Correlations (synchronization between different attributes)
Creating model snapshots
isBatchMode() | ||
Parameter | Type | Description |
Return value | boolean | Function returns true if the script is executed in batch mode. |
Some scripting functions can only be executed in the GUI or in batch mode. The isBatchMode() function can be used to check which mode the FVA-Workbench is in while the script is running, and to react accordingly.
Example
If the script is executed in GUI mode, a dialog queries the user for the directory to be used. In batch mode, the directory in which the model file is located is used.
let filename = "textfile.txt";
let text = "This text will be written to the file";
let directory;
let resultpath;
if (isBatchMode() == true) {
directory = getModelProperty("FOLDER");
} else {
directory = promptDirectory();
}
resultpath = directory+filename;
println("File written: "+resultpath);
writeToFile(resultpath, text, "c", "UTF-8");
getWorkbenchInfo(infoID); | |||||
Parameter | Type | Description | |||
infoID | VERSION - Version number of the FVA-Workbench REVISION - Consecutive revision number FULL- Version number and revision number | ||||
Return value | string | VERSION | e.g. 8.1.0 | ||
REVISION | e.g. 61975 | ||||
FULL | e.g. 8.1.0 61975 |
Example Output version and revision number on console
let version = getWorkbenchInfo(VERSION);
let revision = getWorkbenchInfo(REVISION);
println("FVA-Workbench "+version+" Revision No. "+revision);
getLanguage(); | |||||
Parameter | Type | Description | |||
Return value | string | de | German | ||
en | English |
Example Output different messages after a calculation, depending on the language.
1let status = runCalcMethod("001_SYSTEM_CALCULATION", 1);
2let language = getLanguage();
3let success_message = language == "de" ? "Die Berechnung war erfolgreich" : "Calculation was successful";
4let fail_message = language == "de" ? "Die Berechnung ist fehlgeschlagen" : "Calculation failed";
5if (status == 1) {
6 println(success_message);
7 } else {
8 println(fail_message );
}
Executes a system calculation and saves the return value in the status variable. | |
Queries the current language of the FVA-Workbench and saves the return value in the language variable. | |
Writes the message to be output after a successful calculation to the success_message variable. If the value in the language variable is "en" the English string is used, and vice-versa. | |
As with the success message, the message to be output after a failed calculation is written to the fail_message variable. | |
If the return value in the status variable = 1, then: | |
The success message is output to the scripting console | |
Otherwise: | |
The failure message is output |
This function returns all messages for an executed calculation as a JSON object.
getNotifications(NotificationType); | |||
Parameter | Type | Description | |
NotificationType | String | ALL | All messages |
ERROR | Error messages only | ||
WARNING | Warnings only | ||
INFO | Information messages only | ||
Return value | JSON object | The messages are returned as a nested JSON object. The top level includes information about the calculation that was performed and the calculated component as well as the message itself. Some messages also have a second nested level, which may contain information about the relevant attribute and its value. |
Example Output all messages for a calculation to the Scripting Monitor.
let notifications = getNotifications("ALL");
for(i = 0; i < notifications.length; i++) {
let notification = notifications[i];
println("Notification #" + i);
println("---------------");
println("Type: " + notification.type);
println("CalcMethodCompId: " + notification.calcMethodCompId);
println("CalcMethodCompName: " + notification.calcMethodCompName);
println("CalcMethodId: " + notification.calcMethodId);
println("CalcMethodName: " + notification.calcMethodName);
println("CalcStepName: " + notification.calcStepName);
println("Message: " + notification.message);
println(" ");
let items = notification.items;
for(j = 0; j < items.length; j++) {
let notificationItem = items[j];
println(" Notification Item #" + j);
println(" ---------------------");
println(" AttrId: " + notificationItem.attrId);
println(" CompId: " + notificationItem.compId);
println(" Message: " + notificationItem.message);
println(" Value: " + notificationItem.value);
println(" ");
}
println(" ");
}
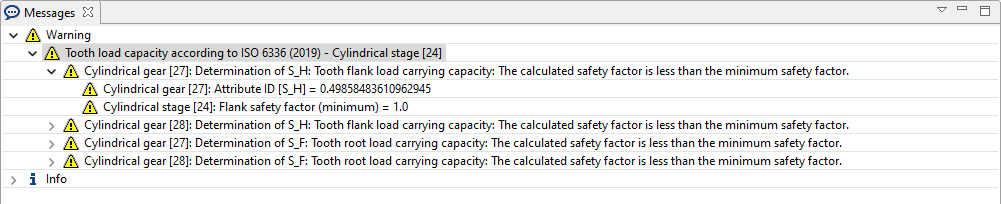
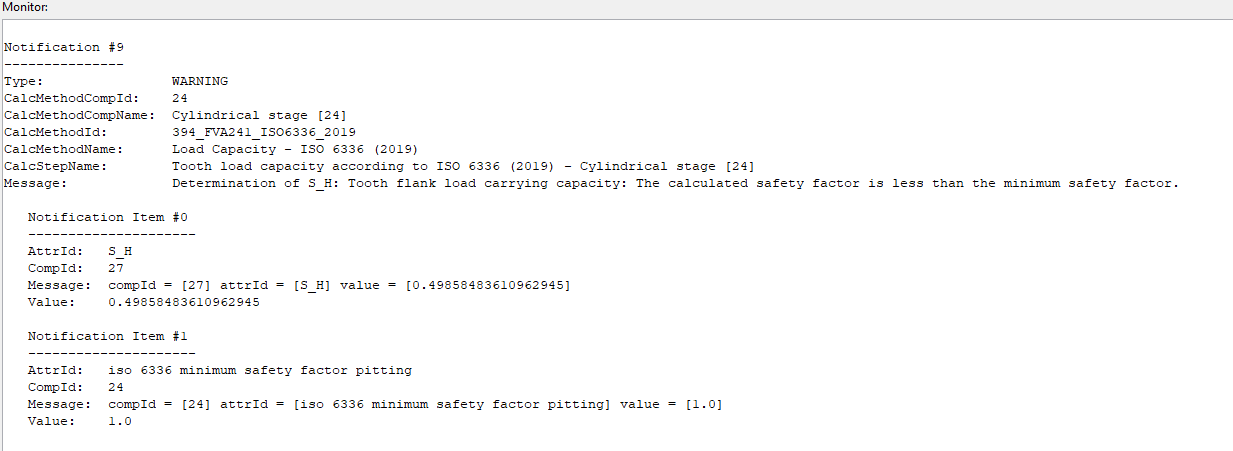
Comparison of the Messages window in the user interface with the script output in the Scripting Monitor.
get(url) | |||||||||||||
Parameter | Type | Description | |||||||||||
url | string | URL of the server from which the data is requested, also called the endpoint. | |||||||||||
header | associative array | Contains metadata and information that the client sends to the server to convey details about the request, the client itself and its preferences. | |||||||||||
Return value (response) | object | The return value is an object from which various properties can be queried:
|
Notice
Further information on handling JSON data can be found here: Reading and writing JSON files
Example 1 Query data from a server
The get request to the endpoint https://postman-echo.com/time/object
returns the current date as a JSON string as a response. The JSON.parse command can be used to convert this string into a JSON object. The elements (properties) in the JSON object can then be accessed via the dot notation.
let response = get('https://postman-echo.com/time/object');
println("Statuscode: " +response.status);
println("Message: " + response.message);
let JSON_object = JSON.parse(response.data);
println("Year: "+JSON_object.years);
println("Month: "+JSON_object.months);
println("Day: "+JSON_object.date);
Example 2 Authentication with username and password
The get request to the endpoint https://postman-echo.com/basic-auth/
requires username and password authentication. If the login was successful, the server returns a JSON string {"authenticated": true}
.
let username = "postman";
let password = "password";
let header = {};
header["Authorization"] = "Basic " + encodeBase64(username + ":" + password);
let response = get("https://postman-echo.com/basic-auth/", header);
println("--- Response ---");
println("Statuscode: " +response.status)
println("Message: " + response.message);
data = JSON.parse(response.data);
println("Login successful: " + data.authenticated);
Example 3 Querying data with URL parameters
The get request to the endpoint https://postman-echo.com/response-headers
can be combined with parameters such as https://postman-echo.com/get?gear1ID=12&gear2ID=22
. In this example, the endpoint returns the parameters given in the response object.
let queryParams = [];
queryParams["param1"] = "Cars";
queryParams["param2"] = "Planes";
println("Endpoint (URL) with appended parameters to which the request is sent: ");
println("https://postman-echo.com/response-headers?" + encodeQueryParams(queryParams));
let response = get("https://postman-echo.com/response-headers?" + encodeQueryParams(queryParams));
println("--- Response ---");
println("Statuscode: " +response.status);
println("Message: " + response.message);
println("Payload: " +response.data);
post(url, header, data) | |||||||||||||
Parameter | Type | Description | |||||||||||
url | string | URL of the server from which the data is requested, also known as the endpoint. | |||||||||||
body | Data sent to the server. | ||||||||||||
header | object | Contains metadata and information that the client sends to the server to convey details about the request, the client itself and its preferences. | |||||||||||
Return value (response) | object | The return value is an object from which various properties can be queried:
|
Example Send data to a server
The post request to the endpoint https://postman-echo.com/post
sends data to the server. For this sample server, the data sent is returned as a response. The data returned by the server depends on the particular implementation of the server. Authentication with username and password is analogous to the get request.
let username = "user";
let password = "pass"
let header = {};
header["Authorization"] = "Basic " + encodeBase64(username + ":" + password);
let content = {"cylindrial_stage": {"helix_angle": 8, "center_distance": 125, "gear1": {"number_of_teeth": 22, "gear_width": 30}, "gear2": {"number_of_teeth": 30, "gear_width": 25}}};
let response = post('https://postman-echo.com/post', content, header);
println("--- Response ---");
println("Statuscode: "+response.status);
println("Message: "+response.message);
let result_object = JSON.parse(response.data);
println("Center distance: "+result_object.data.cylindrial_stage.center_distance);
println("Tooth width gear 1: "+result_object.data.cylindrial_stage.gear1.gear_width);
The following HTTP request methods are supported in the scripting of the FVA-Workbench:
Method | Description |
---|---|
get | The get request is used to read/retrieve data from a web server. It returns an HTTP status code of 200 (OK) if the data was successfully retrieved from the server. |
head | The head request is similar to the get request. However, only the meta information in the header is returned here, not the data. |
post | The post request is used to send data to the server. If delivery is successful, an HTTP status code of 201 is returned. |
put | A put request is used to change the data on the server. It replaces all content at a given point with the data that is passed. If there is no corresponding data, it will be created. |
del (delete) | A del request is used to delete data on the server. |
Examples
let data = {};
let header = {};
let headerForm = {};
let user = "user";
let pwd = "password";
header["Authentication"] = "basic " + encodeBase64(user)+ ":" + encodeBase64(pwd);
headerForm["Content-Type"] = "application/x-www-form-urlencoded";
let queryParams = [];
queryParams["param1"] = "cars";
queryParams["param2"] = "planes";
get("https://myServer.com/?" + encodeQueryParams(queryParams), header);
head("https://myServer.com/", data, header);
post("https://myServer.com/", encodeQueryParams(queryParams), headerForm);
put("https://myServer.com/", data, header);
del("https://myServer.com/", data, header);
get("https://myServer.com/");
head("https://myServer.com/", data);
post("https://myServer.com/", data);
put("https://myServer.com/", data);
del("https://myServer.com/", data);
encodeBase64(string) | |||
Parameter | Type | Description | |
string | string | String | |
Return value | string | Base64 encoded string |
Example 1 Encode the string to Base64 and output it on the Scripting Monitor
let string = "Hello Wold";
let encodedString = encodeBase64(string);
println(encodedString);
decodeBase64(base64string) | |||
Parameter | Type | Description | |
base64string | string | Base64 encoded string | |
Return value | string | Decoded string |
Example 1 Decode a Base64 string and output it on the Scripting Monitor
let base64string = "SGVsbG8gV29ybGQ=";
let decodedString = decodeBase64(base64string);
println(decodedString);
readFileAsBase64(filepath) | |||
Parameter | Type | Description | |
filepath | string | Path + name of the file | |
Return value | string | File as Base64 string |
Example 1 Encode a text file as Base64
let filepath = "c:/example/textfile.txt";
readFileAsBase64(filepath);
decodeBase64ToFile(filepath, base64string) | |||
Parameter | Type | Description | |
filepath | string | Path + name of the file | |
base64string | string | File as a Base64 encoded string | |
Return value | File |
Example Write a base64 encoded string to a text file
let filepath = "c:/example/textfile.txt";
let base64string = "SGVsbG8gV29ybGQ=";
decodeBase64ToFile(filepath, base64string);
encodeQueryParams(queryParameter) | |||
Parameter | Type | Description | |
queryParameter | associative array | Parameters to be encoded | |
Return value | string | Correctly encoded parameters |
Example 1 The variable queryParameter is assigned the parameters a, b and c. The parameters contain invalid characters for URLs. These are replaced with valid characters using the encodeQueryParams() function.
let queryParameter = [];
queryParameter["a"] = "No Spaces";
queryParameter["b"] = "No/Slashes";
queryParameter["c"] = "No ä,ö,ß";
println(encodeQueryParams(queryParameter));
Output of the encoded parameters to the scripting console
a=No+Spaces&b=No%2FSlashes&c=No+%C3%A4%2C%C3%B6%2C%C3%9F