Model snapshots in scripting
From FVA-Workbench version 7.1, model snapshots can be created, named, and deleted in scripting. It is also possible to read attribute values from model snapshots and create (comparison) reports.
Model snapshots include all input and output data, which makes it possible to separate calculations and data evaluation.
Notice
By default, the FVA-Workbench creates a model snapshot after each calculation. However, this is not always desirable when creating model snapshots via scripting. Automatic creation of model snapshots can be deactivated in the reporting preferences (1). The startTransactionMode() function can also be used to deactivate automatic creation of model snapshots while the script is run.
By default, the maximum number of "Current model snapshots" is set to '10' in the reporting preferences. If the script needs to create additional model snapshots, this must be changed in the settings (2). Model snapshots can also be moved directly to the "Saved model snapshots" list after creation using the snapshot.store() function. This list is not limited.
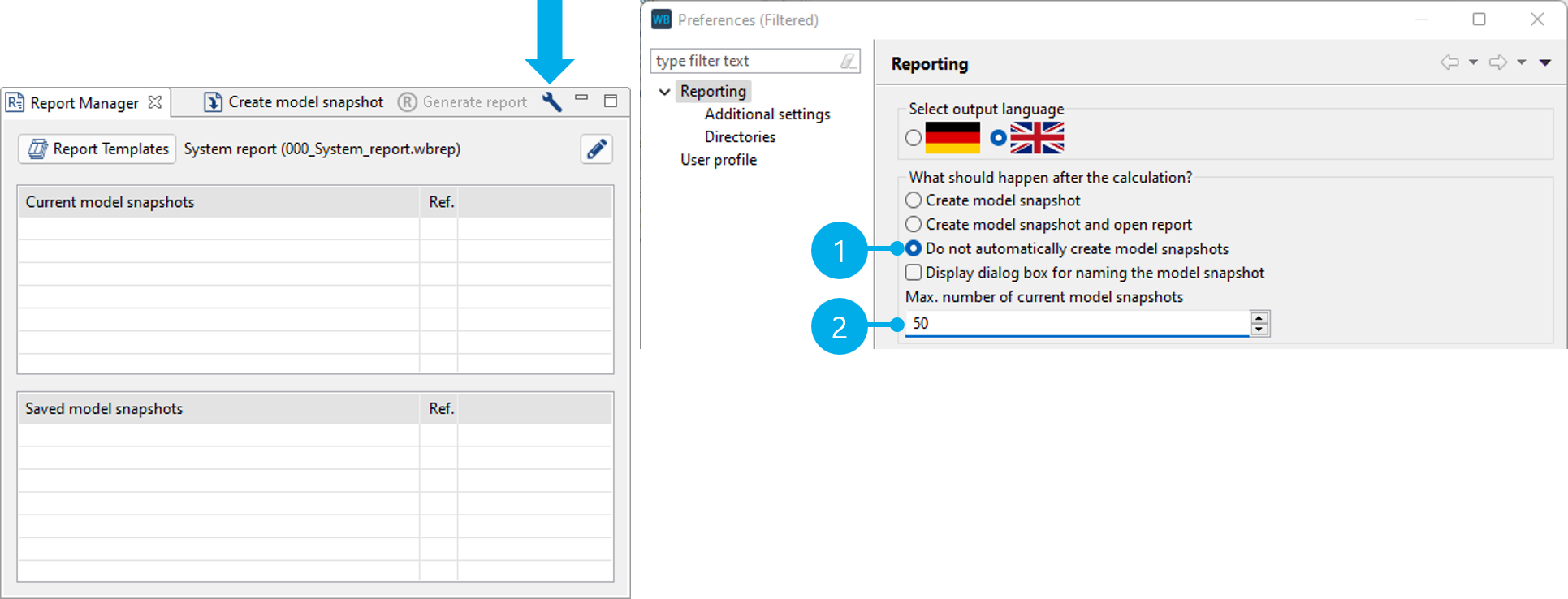
The following functions are available:
snapshotFactory.createSnapshot("title"); | Creates a new model snapshot with the specified title. |
snapshotFactory.getCurrentSnapshots(); | Returns an array with all model snapshots in the "Current model snapshots" list. |
snapshotFactory.getStoredSnapshots(); | Returns an array with all model snapshots in the "Saved model snapshots" list. |
snapshot.getTitle(); | Returns the title of a model snapshot. |
snapshot.setTitle("MySnapshot"); | Sets the title of a model snapshot. |
snapshot.store(); | Moves a model snapshot to the "Saved model snapshots" list. |
snapshot.delete(); | Deletes a model snapshot. |
snapshot.getAttribute(attributeID, componentID); | Delivers the value of an attribute in a model snapshot. |
snapshot.generateReport(templatePath, outputPath, options); | Creates a report from a model snapshot. The parameters correspond to the standard generateReport() function. |
snapshotFactory.compareSnapshots(snapshot1, snapshot2, templatePath, outputPath, options); | Creates a comparison report between two model snapshots. |
Example script
The following script increases the helix angle of a cylindrical gear by 1° in each iteration, runs a system calculation (including gear excitation), and then creates a model snapshot. Then, the transmission error value and the angle value are read from each model snapshot and output to the scripting monitor. Subsequently, an HTML comparison report is generated between the snapshot with the smallest and the snapshot with the largest transmission error.
let reportOutputPath = "c:\\results\\comparison_report.html"; let reportTemplatePath = "c:\\templates\\myTemplate.wbrep"; let reportOptions = {compactView: false, language: "de", completeTree: false, notifications: true, navigationBar: true}; let stored_snapshots = snapshotFactory.getStoredSnapshots(); for(i = 0; i <= stored_snapshots.length-1; i++){ stored_snapshots[i].delete(); } let gearUnitID = 1; let stageID = getCompByType("cylindrical_mesh")[0]; let gearID = getCompByType("cylindrical_gear")[0]; let startAngel = 5; let endAngel = 10; let trans_error_max_array = []; setAttr("gta_switch_deactivate_all_calculations", gearUnitID, true, EDAT); setAttr("gta_switch_3d_load_distribution_analytical", gearUnitID, true, EDAT); setAttr("gta_switch_3d_force_excitation", gearUnitID, true, EDAT); for(let angle = startAngel; angle <= endAngel; angle++){ setAttr("helix angle reference diameter", gearID, angle, EDAT); startTransactionMode(); runCalcMethod("001_SYSTEM_CALCULATION", gearUnitID); endTransactionMode(); let snapshot = snapshotFactory.createSnapshot("Angle: "+angle); snapshot.store(); } stored_snapshots = snapshotFactory.getStoredSnapshots(); for(i = 0; i <= stored_snapshots.length-1; i++){ let helix_angle = stored_snapshots[i].getAttribute("helix angle reference diameter", gearID); let trans_error_max = stored_snapshots[i].getAttribute("result_transmission_error_maximum", stageID); trans_error_max_array.push(trans_error_max); println("Transmission Error"+"("+helix_angle+"°): "+trans_error_max+" µm"); } let maxErrorValue = Math.max.apply(null, trans_error_max_array); let minErrorValue = Math.min.apply(null, trans_error_max_array); let index_maxError = trans_error_max_array.indexOf(maxErrorValue); let index_minError = trans_error_max_array.indexOf(minErrorValue); println("Snapshot '"+stored_snapshots[index_maxError].getTitle()+"' has the largest transmission error ("+maxErrorValue+")"); println("Snapshot '"+stored_snapshots[index_minError].getTitle()+"' has the smallest transmission error ("+minErrorValue+")"); snapshotFactory.compareSnapshots(stored_snapshots[index_minError], stored_snapshots[index_maxError], reportTemplatePath, reportOutputPath, reportOptions);